Unraveling the Python SystemExit Exception: A Comprehensive Insight and Practical Examples
July 2024: Enhance your computer’s performance and eliminate errors with this cutting-edge optimization software. Download it at this link
- Click here to download and install the optimization software.
- Initiate a comprehensive system scan.
- Allow the software to automatically fix and repair your system.
Exception Context and Inheriting from Built-in Exceptions
When working with exceptions in Python, it’s important to understand exception context and how to inherit from built-in exceptions.
To create a custom exception class that inherits from a built-in exception, follow these steps:
1. Define your custom exception class by subclassing from the desired built-in exception class.
2. Implement any additional functionality or behavior required for your specific use case.
3. Use the custom exception class in your code to handle specific error conditions.
When raising an exception, it’s also possible to provide additional context using the `__cause__` attribute. This can be helpful for debugging purposes and providing more information about the error.
Remember to handle exceptions appropriately in your code using a `try` statement and handle specific exception classes using `except` clauses. This ensures that your code can gracefully handle any unexpected situations that may arise.
By understanding exception context and how to inherit from built-in exceptions, you can effectively handle and manage exceptions in your Python code.
Additionally, it automatically fixes missing or corrupt DLL files. For severe issues like the Blue Screen of Death, Fortect repairs the causes and identifies malfunctioning hardware. It also assists with OS recovery by restoring vital system files without affecting user data.
Base Classes and Concrete Exceptions
Python SystemExit Exception Explained and Examples
Base Classes and Concrete Exceptions
Base Class | Concrete Exception | Description |
---|---|---|
BaseException | SystemExit | Indicates an exit request from the program. |
BaseException | KeyboardInterrupt | Indicates an interrupt signal from the keyboard. |
BaseException | Exception | Base class for all exceptions. |
OS Exceptions and Warnings
When working with Python’s SystemExit exception, it is important to understand the various exceptions and warnings that can occur. These exceptions and warnings provide valuable information about the execution of your code and can help you identify and resolve any issues that may arise.
In Python, exceptions are raised when an error or unexpected condition occurs during the execution of a program. The SystemExit exception is a special exception that is raised when the program is terminated by calling the exit() function or when the interpreter is asked to terminate by the operating system.
When handling the SystemExit exception, it is important to note that it is a subclass of the BaseException class, which means that it can be caught and handled just like any other exception. Additionally, the SystemExit exception has a few attributes that can provide additional information about the exception, such as the code attribute, which represents the exit status code.
By understanding the different exceptions and warnings associated with the SystemExit exception, you can effectively handle and troubleshoot any issues that may arise during the execution of your Python code.
python
import sys
def some_function():
try:
# Perform some operation or computation
result = 10 / 0
except ZeroDivisionError:
print("An error occurred: Division by zero.")
sys.exit(1)
some_function()
print("This line will not be reached if sys.exit() is called.")
In this example, the `some_function()` attempts to perform a division by zero, which raises a `ZeroDivisionError`. Inside the `except` block, `sys.exit(1)` is called, which causes the program to terminate with a non-zero exit code. The exit code of `1` indicates an abnormal termination due to the error.
Exception Groups and Hierarchy
- Check for the presence of a SystemExit exception in the code.
- If a SystemExit exception is present, identify the specific code block or section where it is being raised.
- Verify if the SystemExit exception is being used intentionally for program termination or if it is unintentional.
- If the SystemExit exception is intentional, ensure that it is being used appropriately and for the intended purpose.
- If the SystemExit exception is unintentional, evaluate the surrounding code to identify any potential errors or issues that might be causing the exception to be raised.
- Review the exception hierarchy to understand the relationship between the SystemExit exception and other related exceptions.
- Identify any exception groups or categories to which the SystemExit exception belongs.
- Analyze how the exception groups and hierarchy are being utilized in the code to handle different types of exceptions.
- If necessary, make modifications to the exception handling logic to ensure proper handling of the SystemExit exception and other related exceptions.
- Consider implementing additional exception handling mechanisms, such as logging or error reporting, to provide better visibility into the occurrence of SystemExit exceptions.
- Test the modified code to ensure that the exception groups and hierarchy are functioning as expected and that the SystemExit exception is handled appropriately.
It is important to use the System.exit() method cautiously and only when necessary in order to gracefully terminate a program and avoid any unexpected consequences. Download this tool to run a scan
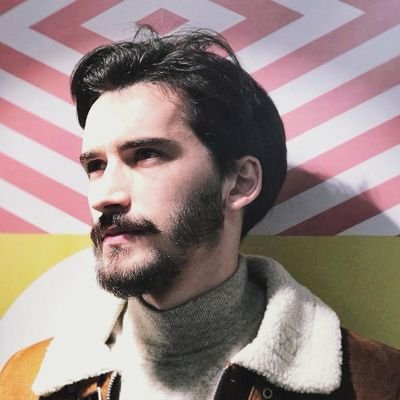