Having trouble with the Discord Squirrel error? Let’s explore effective fixes to resolve this issue and get your Discord up and running smoothly again.
Important
Fortect can help address the discord squirrel error by repairing damaged system files and faulty settings.
Download Now
Clear Discord cache: One common solution to the Discord squirrel error is to clear the app’s cache. This can be done by going to Discord settings, selecting “Appearance,” and then clicking on “Clear Cache.” Restart Discord after clearing the cache to see if the error is resolved.
Troubleshooting Discord installation on Windows 10
If you are facing issues with installing Discord on Windows 10 and encountering a Squirrel error, there are a few troubleshooting steps you can take to resolve the problem.
First, try clearing your browser’s cache and cookies. This can help resolve any conflicts that may be preventing the installation process from completing successfully.
If that doesn’t work, you can try downloading the Discord app from the official website instead of using the Windows Store. Sometimes, the app store version may have compatibility issues with certain systems.
Another option is to run Discord as an administrator. Right-click on the Discord shortcut or the executable file, select “Run as administrator”, and see if that helps resolve the Squirrel error.
If none of these steps work, you can also try uninstalling any previous versions of Discord that may be conflicting with the installation. Use the “Add or Remove Programs” feature in the Windows settings to uninstall Discord completely, then try reinstalling it.
Stop the Discord process
1. Press Ctrl + Shift + Esc to open the Task Manager.
2. In the Processes tab, locate the Discord process.
3. Right-click on the Discord process and select “End Task”.
4. Confirm the action by clicking “End Process”.
By stopping the Discord process, you can resolve the Discord Squirrel error and regain control over your computer.
Please note that this solution is specific to Windows operating systems. If you are using a different operating system, consult the appropriate documentation for instructions on stopping processes.
Uninstall previous Discord app
To fix the Discord Squirrel Error, you need to uninstall the previous Discord app. Follow these steps to remove the app completely from your device:
1. Close Discord and all its related processes running in the background.
2. Open the Start menu and search for “Control Panel.”
3. Click on “Control Panel” to open it.
4. In the Control Panel, navigate to “Programs” or “Programs and Features.”
5. Find “Discord” in the list of installed programs.
6. Right-click on “Discord” and select “Uninstall” or “Remove.”
7. Follow the on-screen instructions to complete the uninstallation process.
8. Once the previous Discord app is uninstalled, you can proceed to reinstall the latest version.
Make sure to remove any remaining files or folders related to Discord if prompted during the uninstallation process.
python
import discord
from discord.ext import commands
intents = discord.Intents.default()
intents.typing = False
intents.presences = False
# Create a bot instance
bot = commands.Bot(command_prefix=’!’, intents=intents)
# Event triggered when the bot is ready
@bot.event
async def on_ready():
print(f’Logged in as {bot.user.name}’)
# Event triggered when an error occurs
@bot.event
async def on_command_error(ctx, error):
if isinstance(error, commands.CommandNotFound):
await ctx.send(“Command not found.”)
elif isinstance(error, commands.MissingRequiredArgument):
await ctx.send(“Missing arguments.”)
else:
await ctx.send(“An error occurred.”)
# Command example
@bot.command()
async def greet(ctx):
await ctx.send(“Hello!”)
# Run the bot
bot.run(‘YOUR_BOT_TOKEN’)
This code demonstrates a simple Discord bot using the discord.py library. It sets up basic error handling for common errors that could occur during command invocation.
Remove leftover files
1. Close Discord completely by right-clicking on the taskbar icon and selecting “Close Discord.”
2. Press the Windows key + R to open the Run dialog box. Type “%appdata%” (without quotes) and press Enter.
3. Locate the Discord folder in the Roaming directory and delete it. This will remove any remaining files associated with Discord.
4. Press the Windows key + R again and type “%localappdata%” (without quotes) in the Run dialog box. Press Enter.
5. Find the Discord folder in the Local directory and delete it as well. This ensures that all files related to Discord are removed.
6. Restart your computer to ensure that any lingering processes are terminated.
7. Download and reinstall Discord from the official website or app store to get a fresh installation.
Run installation as an Administrator
To run the installation of Discord as an Administrator, follow these steps:
1. Right-click on the Discord installation file.
2. From the context menu, select “Run as administrator”.
3. If prompted by User Account Control, click “Yes” to continue.
4. The installation process will now run with elevated privileges, allowing it to make necessary changes to your system.
By running the installation as an Administrator, you ensure that Discord has the necessary permissions to install and function correctly on your computer.
Remember to always exercise caution when running applications with elevated privileges, and only do so when necessary.
If you encounter any further issues or errors, please refer to the relevant troubleshooting articles or contact Discord support for assistance.
Uninstall Windows update
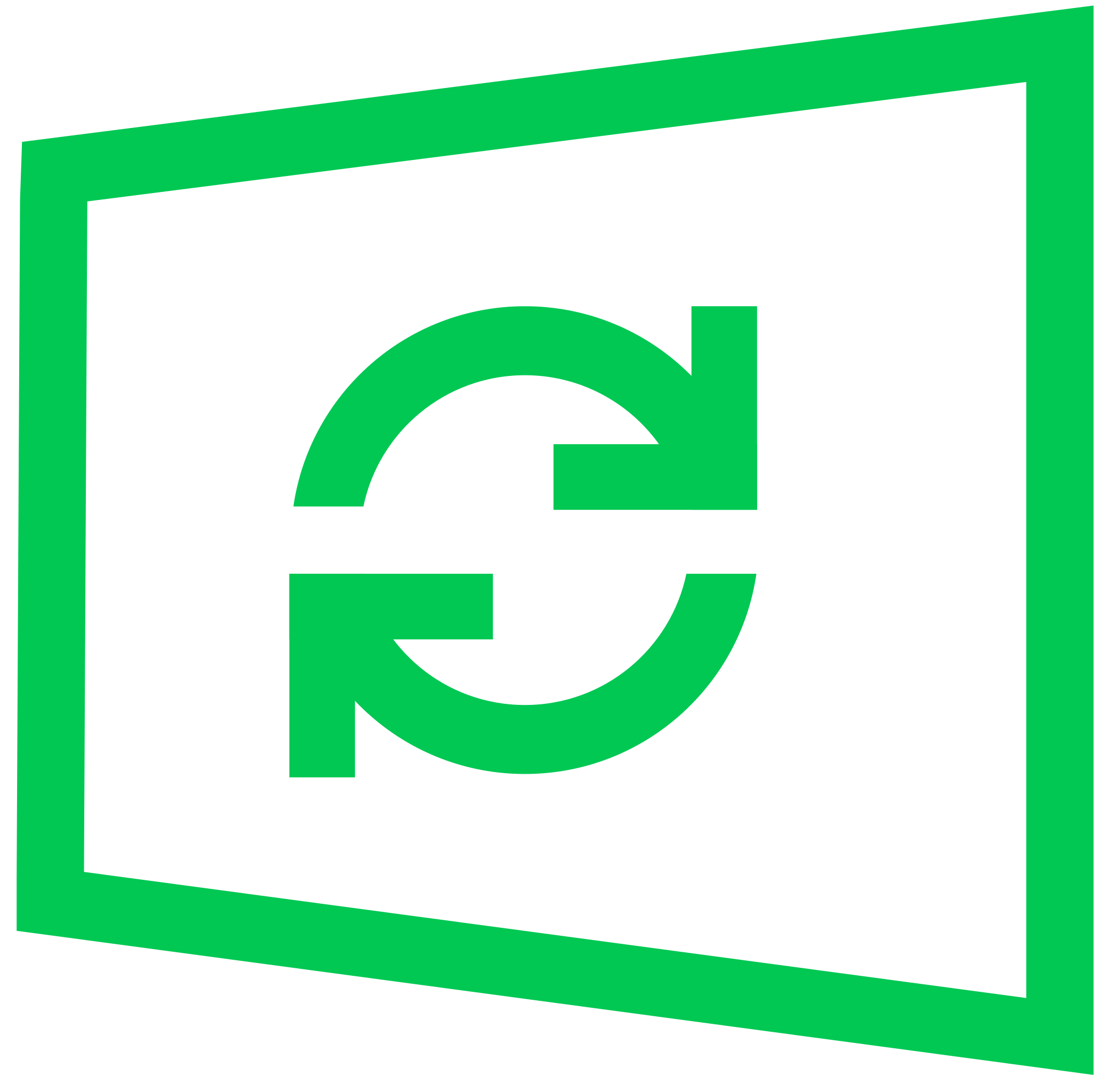
To uninstall a Windows update, follow these steps:
1. Press the Windows key and type “Control Panel”. Open the Control Panel app.
2. In the Control Panel, select “Programs” and then click on “Programs and Features”.
3. On the left-hand side, click on “View installed updates”.
4. Scroll through the list of installed updates and locate the Windows update you want to uninstall.
5. Right-click on the update and select “Uninstall” from the context menu.
6. Follow the on-screen prompts to complete the uninstallation process.
Please note that uninstalling a Windows update may cause certain features or applications to stop working properly. It is recommended to only uninstall updates if absolutely necessary and to consult with a technical expert if you are unsure.
For more information on managing Windows updates and troubleshooting Windows errors, visit the Microsoft Support website.
Disable Discord in Task Manager
1. Press Ctrl+Shift+Esc to open Task Manager.
2. Go to the “Processes” or “Details” tab.
3. Look for any Discord-related processes, such as “Discord.exe” or “DiscordPTB.exe”.
4. Right-click on the process and select End Task.
5. Confirm the action if prompted.
6. Discord will be disabled and no longer running on your computer.
Remember to close any other Discord-related processes if they are still running. This should resolve the Discord Squirrel error.
If you continue to experience issues, try restarting your computer and launching Discord again.
Delete Discord folders
If you’re experiencing the Discord Squirrel Error and need to fix it, one troubleshooting step you can take is deleting Discord folders. This can help resolve any issues related to corrupted files or settings.
To delete Discord folders, follow these steps:
- Exit Discord: Make sure Discord is completely closed. You can do this by right-clicking the Discord icon in the system tray and selecting “Quit Discord”.
- Delete Discord folders: Open the File Explorer on your computer and navigate to the following locations, deleting any Discord folders you find along the way:
- %AppData%\Discord
- %LocalAppData%\Discord
- %LocalAppData%\DiscordSetup
- Restart your computer: After deleting the Discord folders, restart your computer to ensure all changes take effect.
- Reinstall Discord: Finally, download and reinstall Discord from the official website or app store to get a fresh installation.
This process can help resolve various issues, including the Discord Squirrel Error. However, please note that deleting Discord folders will remove your saved settings, so you may need to reconfigure them after reinstalling the application.
If you continue to encounter the Discord Squirrel Error or need further assistance, consider reaching out to Discord support for additional guidance.
Update available device drivers
1. Press the Windows key + X and select Device Manager.
2. Expand the category of the device with the error.
3. Right-click on the device and select Update driver.
4. Choose the option to search automatically for updated driver software.
5. Wait for Windows to search and install the latest driver.
Try installing a different version of Discord
1. Uninstall Discord from your computer.
2. Go to the official Discord website and download a different version of the app.
3. Once the new version is downloaded, run the installation file.
4. Follow the on-screen instructions to install Discord on your computer.
5. Launch the newly installed version of Discord and see if the Squirrel Error persists.
Temporarily disable antivirus
If you are encountering the Discord Squirrel error, disabling your antivirus temporarily might help resolve the issue. Here’s how you can do it:
1. Locate the antivirus software icon in your system tray or taskbar.
2. Right-click on the icon to open the antivirus software interface.
3. Look for an option that allows you to disable or pause the antivirus protection.
4. Click on that option to temporarily turn off the antivirus.
5. Launch Discord again and check if the Squirrel error persists.
Updated: July 2025
Fortect can help with certain aspects of the Discord squirrel error. If the error is caused by damaged system files or faulty settings, Fortect can repair them to resolve the issue.
However, if the error is not related to these specific issues, Fortect may not be able to fix it. It is always recommended to consult with technical support for further assistance.
Turn off Windows Firewall
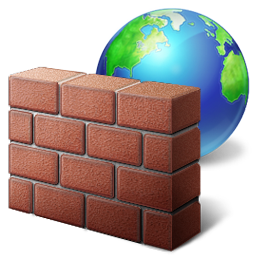
1. Click the Start button and type “Windows Defender Firewall” in the search bar.
2. Select “Windows Defender Firewall” from the search results.
3. In the left navigation pane, click on “Turn Windows Defender Firewall on or off.”
4. Select the option “Turn off Windows Defender Firewall (not recommended)” for both private and public networks.
5. Click “OK” to save the changes.
Methods to fix Discord installation failure
- Open the Control Panel on your computer.
- Select Programs or Add/Remove Programs (depending on your operating system).
- Locate Discord in the list of installed programs.
- Click on Uninstall or Remove to uninstall Discord.
- Go to the official Discord website and download the latest version of Discord.
- Run the installer and follow the on-screen instructions to install Discord again.
- Launch Discord and check if the Squirrel Error is resolved.
Method 2: Clear AppData
- Press Win + R on your keyboard to open the Run dialog box.
- Type %AppData% and press Enter.
- Locate the Discord folder and delete it.
- Press Win + R again to open the Run dialog box.
- Type %LocalAppData% and press Enter.
- Locate the Discord folder and delete it.
- Restart your computer and reinstall Discord from the official website.
- Launch Discord and see if the Squirrel Error is resolved.
Method 3: Use Command Prompt
- Press Win + R on your keyboard to open the Run dialog box.
- Type cmd and press Enter to open Command Prompt.
- Copy and paste the following command into Command Prompt: %LocalAppData%\Discord\Update.exe –uninstall
- Press Enter to execute the command and uninstall Discord.
- Go to the official Discord website and download the latest version of Discord.
- Run the installer and follow the on-screen instructions to install Discord again.
- Launch Discord and check if the Squirrel Error is resolved.
Run installer as an administrator
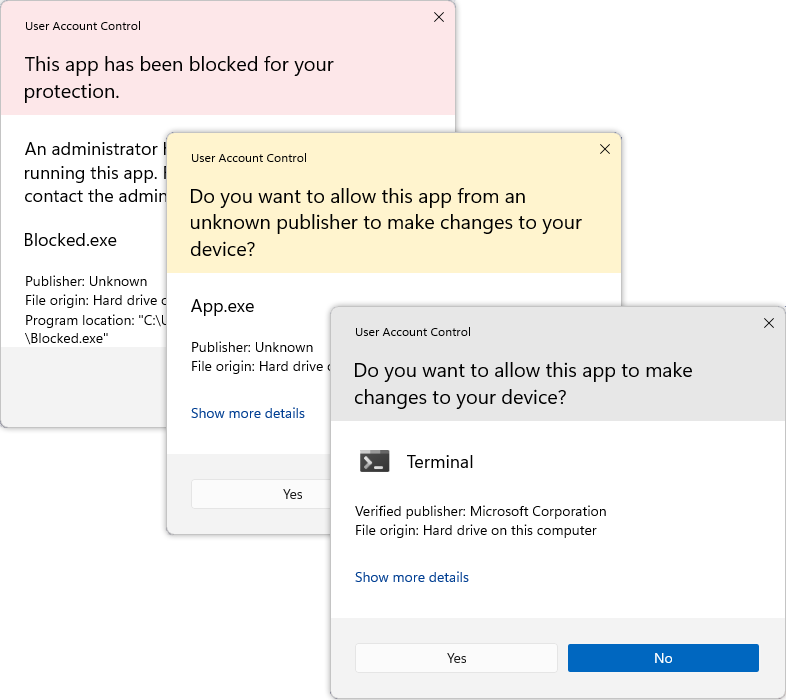
To fix the Discord Squirrel Error, try running the installer as an administrator. Right-click on the installer file and select “Run as administrator” from the context menu. This will give the installer the necessary permissions to make changes to your system. If you are still experiencing issues, you may need to temporarily disable your antivirus software and try running the installer again. Don’t forget to re-enable your antivirus after the installation is complete.
Run installer in compatibility mode
To fix the Discord Squirrel Error, you can try running the installer in compatibility mode. Here’s how:
1. Locate the Discord installer file on your computer.
2. Right-click on the installer file and select “Properties” from the context menu.
3. In the Properties window, navigate to the “Compatibility” tab.
4. Check the box that says “Run this program in compatibility mode for:”.
5. From the drop-down menu, select the version of Windows that you want to run the installer in compatibility mode for.
6. Click on “Apply” and then “OK” to save the changes.
7. Double-click on the installer file to run it in compatibility mode.
Running the installer in compatibility mode can help resolve any compatibility issues that may be causing the Discord Squirrel Error. Give it a try and see if it fixes the problem.
If you continue to experience the error, you may need to try other troubleshooting steps or seek further assistance from Discord support.
For more information about Discord and its features, you can visit the official Discord website. They provide comprehensive guides and resources to help you make the most of the platform.
Restart installer through Task Manager
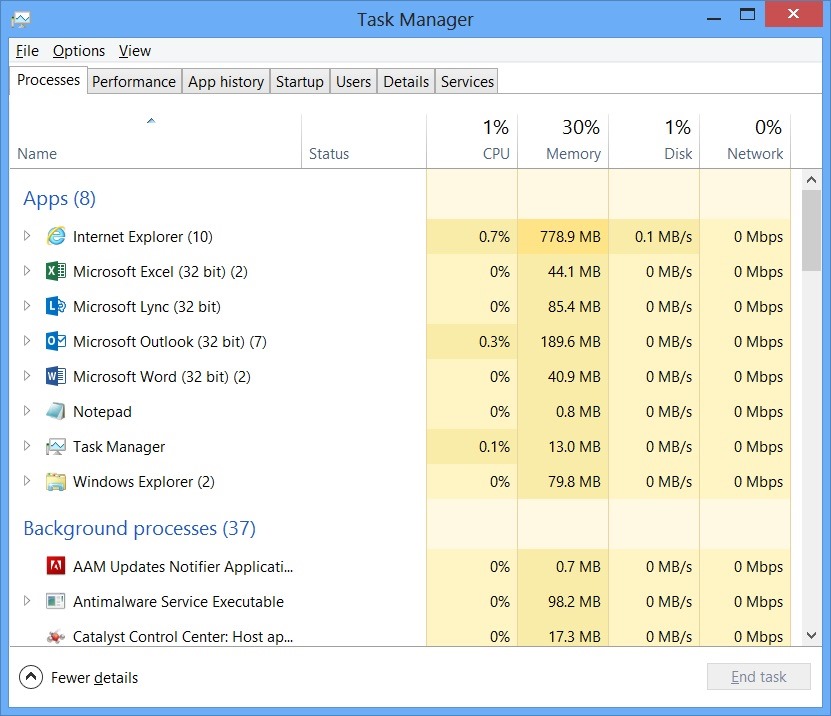
If you encounter the Discord Squirrel Error during installation, you can restart the installer through Task Manager. To do this, follow these steps:
1. Press Ctrl + Shift + Esc to open Task Manager.
2. In the Processes tab, locate and select the Discord installer process.
3. Click on End Task to stop the installer.
4. Close Task Manager and try running the installer again.
If the issue persists, you may need to troubleshoot further or seek additional support.
Completely remove Discord files
1. Close the Discord application and make sure it is not running in the background.
2. Press the Windows key + R to open the Run dialog box.
3. Type “%appdata%” (without the quotes) and press Enter. This will open the AppData folder.
4. Locate the Discord folder and delete it. This will remove all Discord files from your computer.
5. Press the Windows key + R again and type “%localappdata%” (without the quotes) and press Enter. This will open the LocalAppData folder.
6. Locate the Discord folder in this folder as well and delete it.
7. Restart your computer.
After following these steps, you should have completely removed all Discord files from your computer. You can now reinstall Discord and hopefully resolve the Squirrel Error issue.
For more information and troubleshooting steps, you can visit the Discord website or contact their support team.
Stay connected with your friends and communities on Discord without any interruptions!
Common issues with Discord installation on Windows 10
If you are experiencing issues with installing Discord on Windows 10, there are a few common problems that you may encounter. One of these is the Discord Squirrel Error. This error occurs when there is a problem with the Discord installation package.
To fix this error, you can try a few troubleshooting steps. First, make sure that you have a stable internet connection. This is important because Discord requires an internet connection to download and install properly.
Next, you can try clearing your browser cache and cookies. Sometimes, these files can interfere with the installation process. To do this, open your browser settings and navigate to the privacy or history section. From there, you can clear your cache and cookies.
If clearing your browser cache and cookies doesn’t solve the problem, you can try downloading the Discord installation package directly from the Discord website. To do this, go to the Discord website and look for the download page. From there, you can download the installation package and run it on your Windows 10 computer.
If none of these steps work, you can try reaching out to Discord support for further assistance. They may be able to provide additional troubleshooting steps or help you resolve the issue.
Unable to install Discord on Windows 10
If you are encountering the Discord Squirrel Error while trying to install Discord on Windows 10, follow these steps to resolve the issue:
1. Close Discord and any associated processes running in the background.
2. Delete Discord’s app data by navigating to the following location: C:\Users\%USERNAME%\AppData\Roaming\Discord.
3. Delete the Discord installation folder located at C:\Users\%USERNAME%\AppData\Local\Discord.
4. Clear your browser cache and cookies as they may interfere with the installation process.
5. Restart your computer to ensure all changes are applied.
6. Download the latest version of Discord from the official website or Microsoft Store.
7. Install Discord using the downloaded installer and follow the on-screen instructions.
If the issue persists, consider temporarily disabling your antivirus software as it may be blocking the installation. Once Discord is successfully installed, you can re-enable your antivirus software and add Discord to its exception list if necessary.
Discord installation failed on Windows 10
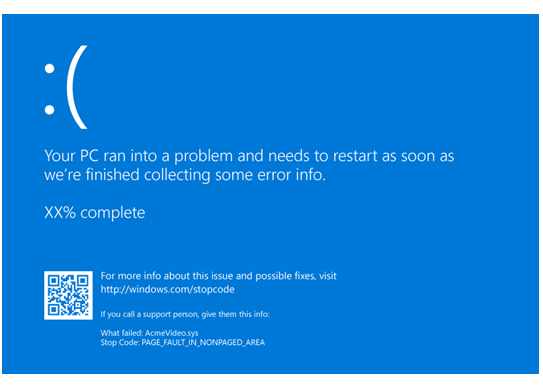
If you encountered a Discord installation failure on Windows 10, you may have encountered the Discord Squirrel Error. To fix this issue, follow these steps:
1. First, ensure that you are running the latest version of Windows 10. Updating your operating system can often resolve compatibility issues.
2. Next, try running the Discord installer as an administrator. Right-click on the installer file and select “Run as administrator” from the context menu.
3. If the above steps did not resolve the issue, try deleting Discord’s app data and cache files. Press Win + R to open the Run dialog, then type %AppData%\Discord and press Enter. Delete all files and folders within this directory.
4. Additionally, delete any remaining Discord files by opening the Run dialog again and typing %LocalAppData%\Discord. Press Enter and delete all files and folders in this directory as well.
5. After deleting the app data and cache files, restart your computer and try reinstalling Discord.
6. If the issue persists, you may need to disable your antivirus or firewall temporarily. Some security software can interfere with the installation process. Remember to re-enable your antivirus and firewall after the installation is complete.
7. Finally, if none of the above solutions worked, you can try using an alternative method to install Discord. Visit the official Discord website and download the installer from there instead of using the Windows Store.
Additional tips and recommendations for Discord installation
- Ensure that your device meets the system requirements for Discord installation.
- Download the latest version of Discord from the official website or a trusted source.
- Disable any antivirus or firewall temporarily, as they might interfere with the installation process.
- Run the Discord installer as an administrator to avoid any permission issues.
- Clear your browser cache and temporary files to ensure a clean installation.
- Use a stable internet connection to prevent any interruptions during the installation.
- Close any unnecessary programs or processes running in the background to free up system resources.
- Restart your device before attempting to install Discord to resolve any conflicting issues.
- Verify that you have enough disk space available for the installation.
- Check for any corrupted or outdated drivers that might cause installation errors and update them.
- Temporarily disable any virtualization software such as VMware or VirtualBox, as they can interfere with Discord installation.
Available in other languages
Discord is available in multiple languages to cater to users worldwide. To change the language settings, follow these steps:
1. Open the Discord application on your device.
2. Click on the gear icon located at the bottom left corner to access User Settings.
3. In the left sidebar, click on “Language”.
4. Select your preferred language from the drop-down menu.
5. Click on “Save Changes” to apply the new language setting.
By changing the language, you can enjoy Discord in a language that suits you best. Whether it’s English, Spanish, French, or any other supported language, Discord has you covered.
Note that changing the language setting will only affect the Discord user interface and not the language of the content shared within servers.
If you encounter any issues or need further assistance, refer to the Discord support documentation or reach out to their customer support team for help.
Recommended articles
1. Understanding the Discord Squirrel Error: Learn about the common causes behind the Discord Squirrel Error and how to troubleshoot it effectively. This article provides step-by-step instructions to help you resolve the issue quickly.
2. Clearing Your Browser’s Cache and Cookies: Sometimes, outdated or corrupted cookies can cause the Discord Squirrel Error. Follow this guide to learn how to clear your browser’s cache and cookies, ensuring a smooth experience on Discord.
3. Updating Discord Mobile App: If you’re encountering the Squirrel Error on your mobile device, it might be due to an outdated version of the Discord app. Discover how to update the app on your iOS or Android device to eliminate the error.
4. QR Code Authentication on Discord: Enhance your account security by enabling QR code authentication on Discord. This feature adds an extra layer of protection to your account and can help prevent future errors or unauthorized access.
5. Advertising Guidelines on Discord: Familiarize yourself with Discord’s advertising guidelines to ensure you’re following the platform’s policies. This article outlines the rules and best practices for advertising on Discord, promoting a positive and engaging community.
6. Exploring Discord’s Privacy Policy: Gain insights into Discord’s privacy policy and understand how your personal information is handled. Knowing your rights and how Discord protects your data is essential for a secure and trustworthy online experience.
7. Efficient Navigation Tips for Discord: Discover handy navigation tips and shortcuts to optimize your Discord experience. This article provides helpful techniques to move swiftly between channels, servers, and DMs, saving you time and improving productivity.
Final thoughts
If you’re encountering the Discord Squirrel Error, we’ve got you covered with some quick and easy fixes. First, try clearing your HTTP cookies as they can sometimes cause issues with the Discord app. If that doesn’t do the trick, consider reinstalling the mobile app to ensure you have the latest version.
Example Message
?
If you encounter a Discord squirrel error, try restarting the application or clearing its cache to resolve the issue. Download this tool to run a scan